This article data science blogthon.
prologue
A web app is an app for exposing a solution or approach to the masses. Creating a model isn’t enough until people use it. Everyone creates her website when it comes to providing solutions. However, you can deploy AI applications using Azure cognitive services. There we will build a simple app for language translation.
system
The first step before developing a project is to remove all prerequisites for project development and its runtime. So, we’ll follow a simple 3-step process to prepare your system to run your code with the necessary libraries and software to help you build a translation app using Azure Cognitive Services.
Step 1) Create an Azure account
You can create an account with Azure for free for 12 months. This account allows you to use most Azure cognitive services for free. As long as you don’t exceed your compute resources before the limit, you won’t be charged. See official Azure. documentation Please follow the instructions.
Step 2) Download Python 3.6+
To create a Flask language translation application, you need Python installed on your system. If you already have Python, you can jump to step 3. Otherwise, you can download python from this official python download page. Link.
Step 3) Install a code editor
It is recommended to use VS code An editor that lets you code in different languages. Apart from VS Code, you can choose other Python IDEs.
Setting up the development environment
We have completed the download and installation part of the project prerequisites, but we need to install certain libraries in our software before we can write any code. First, create a new directory to develop your project in and open the folder in your code editor.
Create a Python virtual environment
It’s always good to develop a new project in a new virtual environment which helps install only the libraries that the project works with. Creating a virtual environment modularizes your application and avoids application versioning issues. Based on your operating system, you can create a virtual environment using the command below.
# Windows # Create the environment python -m venv venv # Activate the environment .venvscriptsactivate
# macOS or Linux # Create the environment python -m venv venv # Activate the environment source ./venv/bin/activate
Install Flask and required libraries
Create a new text file in your working directory and name it requirements.txt. Add the following libraries to the file shown in the snippet below and save.
flask python-dotenv requests
To install all libraries, run the following command:
pip install -r requirements.txt
Create a Flask app
Flask is a Python package used to create static, responsive websites and data apps using Python. Flask provides options for easily routing and referencing different URLs. I hope you are familiar with Flask. See this article if you want an overview.
First, create a landing page for your application. This page displays a form that accepts details from the user. I’m creating a language translation application where users enter text in a form which is the source text and the target language where the results will be displayed. Azure cognitive services can automatically detect the source language. First, we’ll create a simple flask app that routes the application to a home template that contains a form for entering details.
Step 1) Create a core application.
Create a new project folder in your working directory and open that folder in your code editor. Create a new python file and name it app.py. Create a flask app here. Below is a code snippet for creating the default route for the application.
from flask import Flask, request, session, render_template, url_for, redirect app = Flask(__name__) #Add the route @app.route('/', methods=['GET']) def index(): return render_template('index.html')
explanation ~ The import statement contains references to flasks, functions, and modules for building templates like render_template, which is used to render the user to an HTML template at the defined URL.
Step 2) Create HTML Template
Jinja is an HTML-focused template engine for Flask.we use a little bootstrap To make the page look a little cleaner. By using Bootstrap we will use different classes. If you’re not familiar with Bootstrap, don’t worry and focus on the HTML part. Flask templates should be created in a folder named templates.
- Create a new folder named templates where app.py will be kept (in the project folder itself).
- Select the Templates folder and create a new HTML file named index.html and you can follow the code snippet below.
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" integrity="sha384-TX8t27EcRE3e/ihU7zmQxVncDAy5uIKz4rEkgIXeMed4M0jlfIDPvg6uqKI2xXr2" crossorigin="anonymous">
Enter the text you want to translate, select the language,[翻訳]Click.
English
Italian
Japan
Russia
German
Forms are an important part of comprehension and include a text area for users to enter text, a drop-down list for selecting the target language, and a submit button that, when clicked, submits and retrieves details from the translation service. The resulting translated text.
Step 3) Test your application.
Now that the site home page is initialized, it’s time to test it. Follow his two commands below to start the server.
- Set the Flask runtime to development by running the following command: This means that the server will automatically reload whenever you make changes to your Flask app. Run the following command, depending on your operating system:
# Windows set FLASK_ENV=development # Linux/macOS export FLASK_ENV=development
flask run
- Open your application in a browser and navigate to the URL you received when running the command, or visit the default Flask root. http://localhost:5000.
Create a translator service
Azure offers a set of services called Cognitive Services, and the Translation Service is part of the Cognitive Services. Call the translator service like you would create a route for any other endpoint. A key is required to call the translation service. This key will be used each time you call the service to translate text. A good solution for protecting keys is to use dotenv which contains key-value pairs. I don’t want this to be part of the source code.
- Go to Azure Portal,[新しいリソースの作成]Choose.
- In the resource search box, enter translator.
- Select a translator and click Create.Enter the following values in the translator creation form
- Subscription – Subscription.
- In Resources, the group creates a new one and names it Flask-ai.
- Resource Group Region – Choose a name that is close to your location.
- Resource Region – Enter the same value you entered above.
- Name – Any unique name of your choice.
- Pricing Tier – Free F0
- [確認して作成]Choose.[作成]Click and the resource will be created in a few moments.
- go to resource
- [リソース管理]on the left under[キーとエンドポイント]Choose.
- next to key 1[クリップボードにコピー]Choose.
- Write down the text translation and location value.
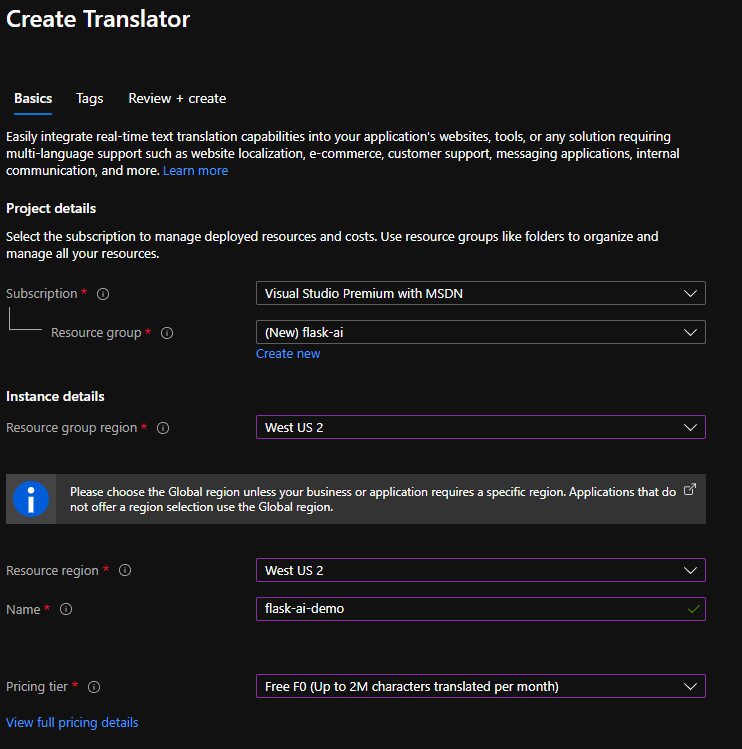
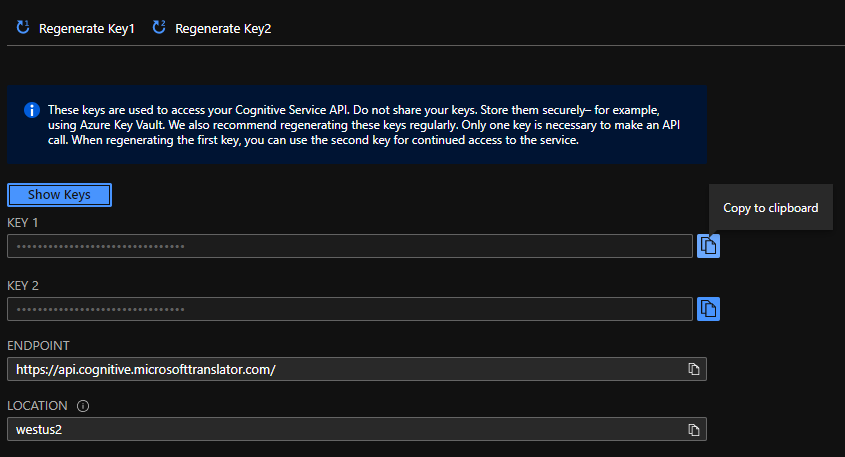
Create .env file
Back in your code editor, create a new file in your project’s root directory and name it .env. The file contains values for keys, endpoints, and locations. After creating the file, paste the following text:
KEY=your_key ENDPOINT=your_endpoint LOCATION=your_location
Paste the key you copied above instead of the key. The endpoint and location must be entered from Azure.
Invoke Translator Service
I created a translation service in the Azure portal by backend-side means. Next, add the necessary logic to add templates and add routing to add translators to your application.
Add code to call the service.
App.py is the main file containing your application code. We need to create a default route so that when the user submits the form, we can access the information and call the translation service. So first you need to import the required modules and load the key pair file.
import json, os, uuid, requests from dotenv import load_dotenv load_dotenv()
Next, create a route that receives the information, calls the translator service, accepts the response from the translator, and displays the translated text to the user.
Read the comments in the code snippet above to understand some of its implications. In a nutshell, here are the steps the code does:
- Read the text entered by the user and the target language.
- Load environment variables stored in the .env file.
- Create a path to call the translation service containing the target language (the source language is automatically identified).
- Create header information including where to send and any IDs.
- Create the text that needs translation.
- Apply a Post request to invoke the translation service.
- Get a JSON response from the server containing the translated text into the target language.
- Using a slice from a JSON object will only get the resulting translated text.
- Display the translated text to the user by rendering it on the results page.
Create a template to display results
In the last step I mentioned rendering the user on the results page to show the translated text. So create an HTML page in the templates folder that will display the translated text using a Jinja template.
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" integrity="sha384-TX8t27EcRE3e/ihU7zmQxVncDAy5uIKz4rEkgIXeMed4M0jlfIDPvg6uqKI2xXr2" crossorigin="anonymous">
result
Original: {{original text}}
Translated text: {{ translated text }}
Target language code: {{ target language }}
test the page
Follow the same steps to start the flask server from the integration template and run commands. Go to localhost:5000, fill out the form and submit.
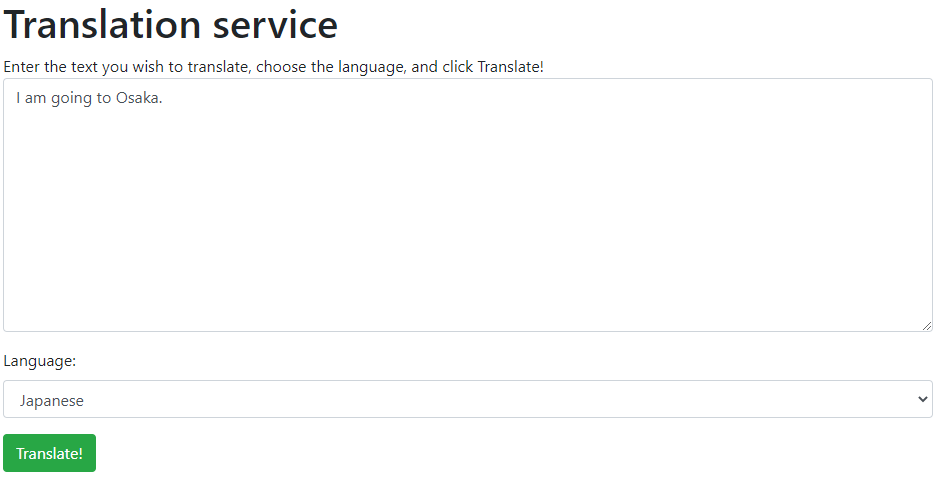
If all goes well, you will get the translated text.
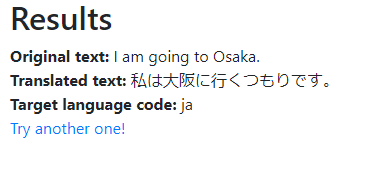
Conclusion
Congrats! You have successfully created an application that translates text from one language to another using Azure Cognitive Services. In practice, languages are context sensitive and computers can’t interpret results the same way humans can, so if you notice results, they won’t be perfect every time you run your application.let’s look at some important point From this article.
- A route is a path to an action, like tapping a button in a mobile app. In our app, a click to submit will perform a specific action.
- Templates let you write core HTML. It also uses a placer to pass information from the server that Jinja’s templating engine to Flask. Therefore, use {{ }} as placeholders to display information.
- Azure cognitive services provide multiple services such as computer vision, speech-to-text, text-to-speech, and text translation.
- Whenever you upload code to a public or open source platform, mark your .env file with git ignores to keep your credentials in the file safe.
Thank-you letter
- We hope that you were able to easily cope with each step described in this article. You can post your questions or connect with me in the comments section below.
- connect with me link in.
- Analytics Vidhya and crazy tech
Media shown in this article are not owned by Analytics Vidhya and are used at the author’s discretion.